12 bit DAC LED
The 12 bit DAC would be very difficult to make on our own with circuit, which is why we are using Adafruit's design. The communication ...
The 12 bit DAC would be very difficult to make on our own with circuit, which is why we are using Adafruit's design. The communication between the DAC and Arduino is handled by software written by Adafruit as well, so we cannot use Scratch to interface with this DAC. The blue wire should attatch to a grounded LED to visualize the sine and triangle waveforms that can be output by the DAC. The full Adafruit code includes different resolutions of the sine waveform, so students can experiment with these waveforms to visualize the choppier to smooth transition as resolution of the sine waveform increases. A delay command in the running loop allows the students to decrease the frequency of the sine wave so the frequency is visible.
As the sine and triangle waveform are running, the students should notice how different the LED behaves from the 2-bit DAC case, when there were only 4 states for the LED to be in. With 12 bits, the LED brightness seems continuous!
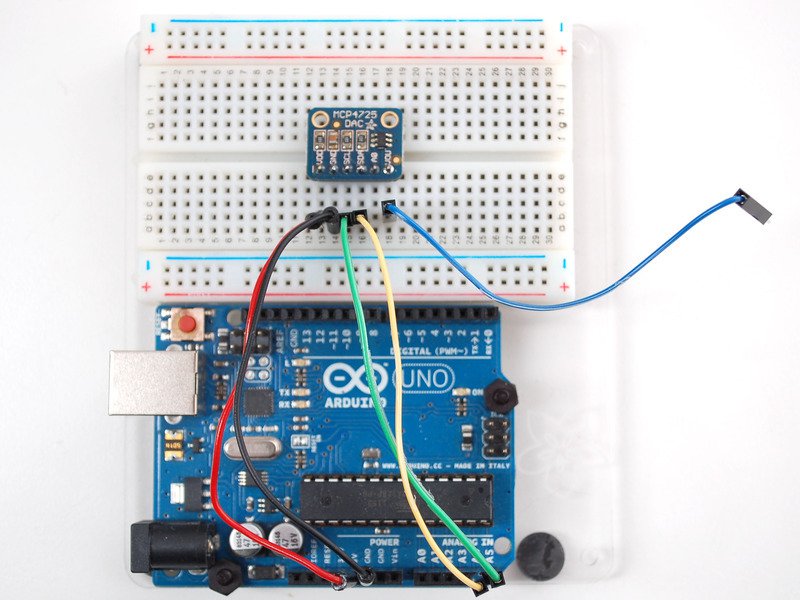
As the sine and triangle waveform are running, the students should notice how different the LED behaves from the 2-bit DAC case, when there were only 4 states for the LED to be in. With 12 bits, the LED brightness seems continuous!
/**************************************************************************/
/*!
@file sinewave.pde
@author Adafruit Industries
@license BSD (see license.txt)
This example will generate a sine wave with the MCP4725 DAC.
This is an example sketch for the Adafruit MCP4725 breakout board
----> http://www.adafruit.com/products/935
Adafruit invests time and resources providing this open source code,
please support Adafruit and open-source hardware by purchasing
products from Adafruit!
*/
/**************************************************************************/
#include <Wire.h>
#include <Adafruit_MCP4725.h>
Adafruit_MCP4725 dac;
// Set this value to 9, 8, 7, 6 or 5 to adjust the resolution
#define DAC_RESOLUTION (8)
/* Note: If flash space is tight a quarter sine wave is enough
to generate full sine and cos waves, but some additional
calculation will be required at each step after the first
quarter wave. */
const PROGMEM uint16_t DACLookup_FullSine_8Bit[256] =
{
2048, 2098, 2148, 2198, 2248, 2298, 2348, 2398,
2447, 2496, 2545, 2594, 2642, 2690, 2737, 2784,
2831, 2877, 2923, 2968, 3013, 3057, 3100, 3143,
3185, 3226, 3267, 3307, 3346, 3385, 3423, 3459,
3495, 3530, 3565, 3598, 3630, 3662, 3692, 3722,
3750, 3777, 3804, 3829, 3853, 3876, 3898, 3919,
3939, 3958, 3975, 3992, 4007, 4021, 4034, 4045,
4056, 4065, 4073, 4080, 4085, 4089, 4093, 4094,
4095, 4094, 4093, 4089, 4085, 4080, 4073, 4065,
4056, 4045, 4034, 4021, 4007, 3992, 3975, 3958,
3939, 3919, 3898, 3876, 3853, 3829, 3804, 3777,
3750, 3722, 3692, 3662, 3630, 3598, 3565, 3530,
3495, 3459, 3423, 3385, 3346, 3307, 3267, 3226,
3185, 3143, 3100, 3057, 3013, 2968, 2923, 2877,
2831, 2784, 2737, 2690, 2642, 2594, 2545, 2496,
2447, 2398, 2348, 2298, 2248, 2198, 2148, 2098,
2048, 1997, 1947, 1897, 1847, 1797, 1747, 1697,
1648, 1599, 1550, 1501, 1453, 1405, 1358, 1311,
1264, 1218, 1172, 1127, 1082, 1038, 995, 952,
910, 869, 828, 788, 749, 710, 672, 636,
600, 565, 530, 497, 465, 433, 403, 373,
345, 318, 291, 266, 242, 219, 197, 176,
156, 137, 120, 103, 88, 74, 61, 50,
39, 30, 22, 15, 10, 6, 2, 1,
0, 1, 2, 6, 10, 15, 22, 30,
39, 50, 61, 74, 88, 103, 120, 137,
156, 176, 197, 219, 242, 266, 291, 318,
345, 373, 403, 433, 465, 497, 530, 565,
600, 636, 672, 710, 749, 788, 828, 869,
910, 952, 995, 1038, 1082, 1127, 1172, 1218,
1264, 1311, 1358, 1405, 1453, 1501, 1550, 1599,
1648, 1697, 1747, 1797, 1847, 1897, 1947, 1997
};
void setup(void) {
Serial.begin(9600);
Serial.println("Hello!");
// For Adafruit MCP4725A1 the address is 0x62 (default) or 0x63 (ADDR pin tied to VCC)
// For MCP4725A0 the address is 0x60 or 0x61
// For MCP4725A2 the address is 0x64 or 0x65
dac.begin(0x62);
Serial.println("Generating a sine wave");
}
void loop(void) {
uint16_t i;
for (i = 0; i < 256; i++)
{
dac.setVoltage(pgm_read_word(&(DACLookup_FullSine_8Bit[i])), false);
#delay()
}
}
1 comments:
Hey mate! If you're looking for a tree lopper around Cairns, book us a quote now! Click for info.
REPLY